5 JavaScript Function Things I Regret Not Knowing Earlier
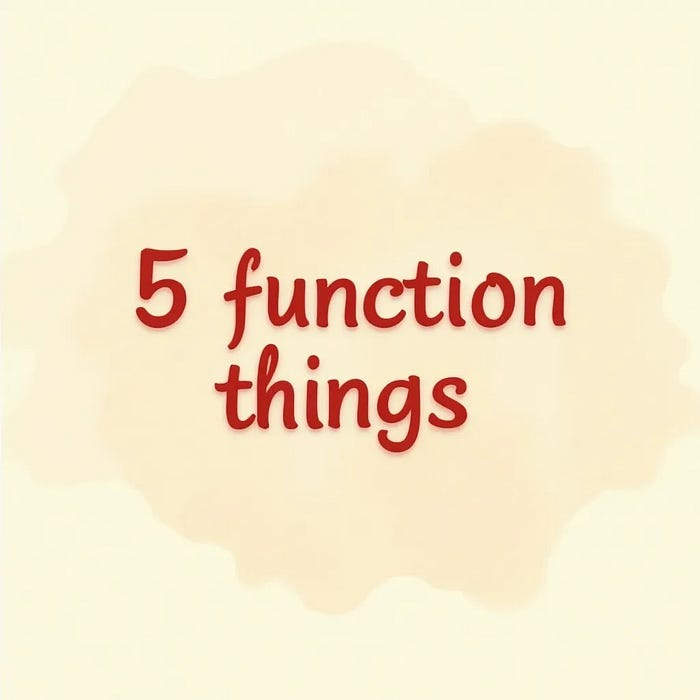
When you work with a certain language for a long time, you can become lazy about learning new things.
Recently, I was working on the login logic for our enterprise application and realized how important it is to understand the details of a programming language to avoid bugs that are difficult to find.
Here are 5 things about JavaScript functions that I wish I had known earlier.
1. Hoisted vs non-hoisted functions
There are three ways to define a function:
The interesting part is what happens when they are called.
Functions declared using the standard function
declaration syntax are hoisted to the top of their scope. This means you can call them before they’re defined in the code, and they’ll still work.
2. Arrow functions and this binding
regularMethod
: This is a regular function. When we callperson.regularMethod()
, thethis
keyword insideregularMethod
refers to theperson
object, sothis.name
outputs"John"
.arrowMethod
: This is an arrow function, which does not have its ownthis
context. Instead, it inheritsthis
from its surrounding lexical scope (the global scope, in this case). Whenperson.arrowMethod()
is called,this
doesn’t refer to theperson
object, sothis.name
isundefined
.
Let’s take a look at another example:
regularMethod
withsetTimeout
: Here,setTimeout
is passed a regular function. In JavaScript, when a regular function is called insidesetTimeout
,this
no longer refers to theperson
object but to the global object (orundefined
in strict mode). This is whythis.name
results inundefined
.regularMethod2
withsetTimeout
: The function insidesetTimeout
is now an arrow function, which doesn’t have its ownthis
context. Instead,this
is lexically bound to thethis
ofarrowMethod
, which isperson
. Therefore,this.name
correctly outputs"John"
.
3. Function parameters with rest parameters
The rest parameter (…
) is useful to gather different kind of values into a single option. I like to use it if to have a more flexible function definition and separate optional parameters from required ones.
4. Higher-Order functions
Higher-order functions are useful to add common functionality (like logging, error handling, or performance testing) to multiple functions.
5. Function composition
Function composition combines multiple functions so each function’s output feeds into the next. This makes complex logic more modular and reusable by breaking it down into smaller, testable parts, ideal for data processing or transformation tasks.