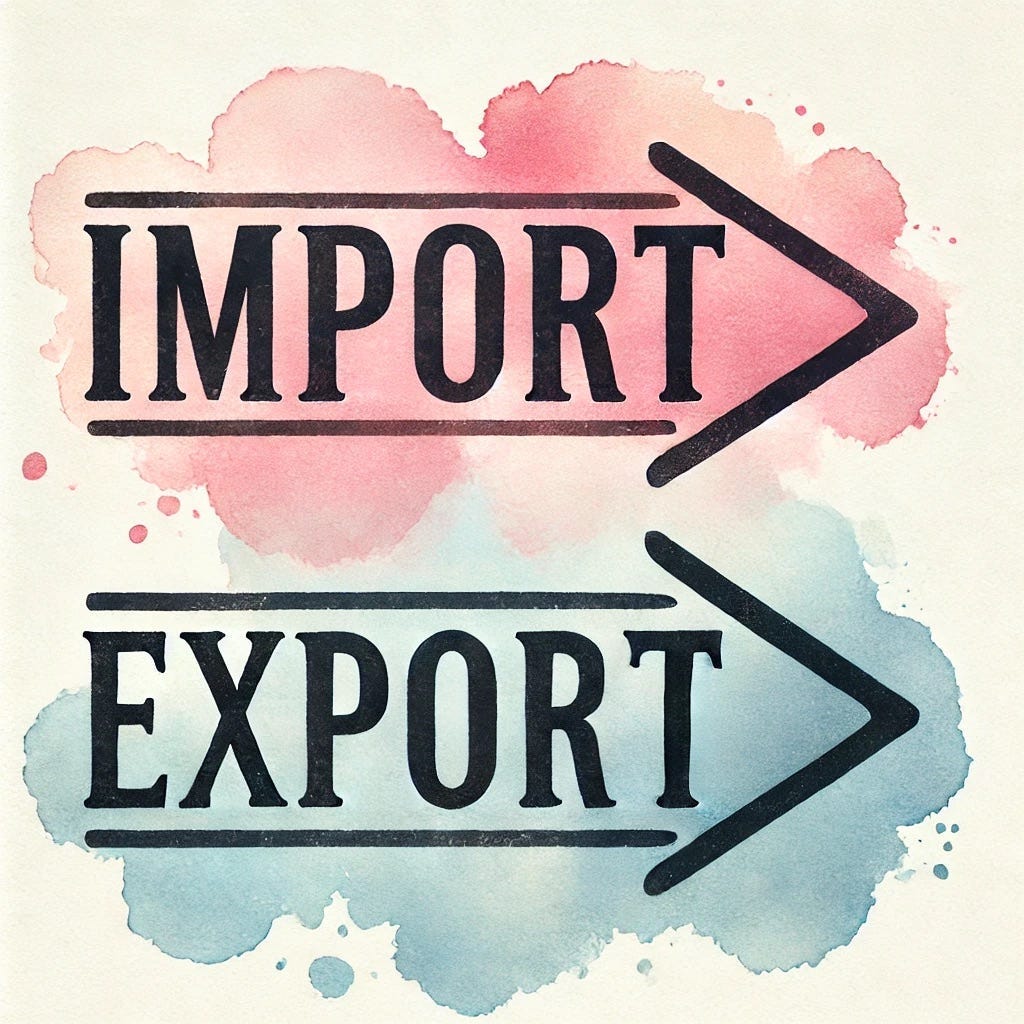
While working on a large-scale B2B application, I learned a few important things about imports and exports in JavaScript.
1) Named Import vs. Default Import
Named import:
With named imports, you explicitly export specific variables, functions, or objects by name. These named exports can be imported selectively, which is useful when you don’t need everything from a module.
Default import:
A module can have only one default export. When using a default export, it can be imported without curly braces and named anything during the import.
2) Namespace Imports
Namespace imports allow you to import everything from a module (both named exports and the default export) as a single object. This is useful when you want to bundle all exports together and access them via a single namespace.
3) Side-Effect Imports
Side-effect imports are used when a module doesn’t export anything, or when you just want to execute code within the module (such as polyfills, global state modifications, or setup code). This type of import doesn’t bind any variables or objects from the module — it simply runs the code inside the module when imported.
4) Dynamic Inputs
Dynamic imports allow you to load a module at runtime, returning a Promise that resolves to the module’s exports. You can dynamically import both named and default exports based on runtime conditions.
5) Use Named Exports for Better Refactorability
In larger projects, it’s better to use named exports. They are easier to refactor and provide better tree-shaking, meaning unused code is removed from the final bundle.
Named exports are also easier to refactor because IDEs can track them more reliably.
6) Use Barrel Files to Simplify Imports
Barrel files re-export modules from a directory, making imports cleaner and more manageable in large projects.
This reduces the number of import paths in consuming files and helps maintain an organized structure. It also improves readability and simplifies refactoring.
7) Follow a Consistent Import Order
A consistent order helps developers to quickly identify external dependencies and local files.
Use a linting rule (e.g., ESLint’s import/order
) to enforce a consistent import order.
8) Avoid Circular Dependencies
Circular dependencies can cause unpredictable behavior, memory leaks, or bugs, especially in complex applications. It’s important to regularly check for them and refactor modules when necessary.
Tools like ESLint’s import/no-cycle
rule can help. At Cresta, we use madge, which runs when we create a PR, to catch circular dependencies early.
9) Use Dynamic Loading with React.lazy
Dynamic imports allow you to split code into smaller chunks and load them only when necessary, improving performance.
Use React.lazy()
with import()
for dynamic imports, especially for loading large components like dashboards, charts, or forms.
This improves performance by loading only the necessary modules when required, leading to faster initial load times and a better user experience.
Learn more:
EchoAPI consistently delivers quick responses, making my API testing incredibly efficient!