You Need to Know These 9 Production-Grade JavaScript Code Styles I’ve Picked Up From Work
Simple changes compound over time to create a cleaner code base.
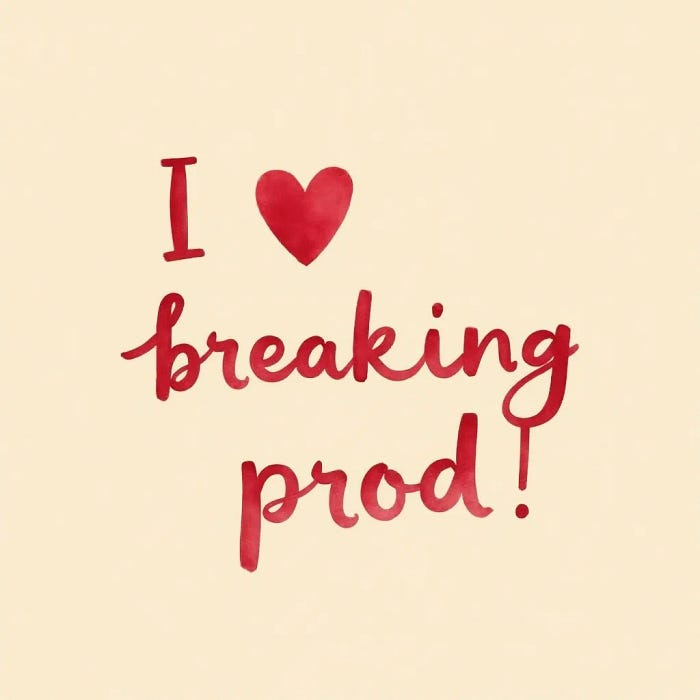
I have noticed that several functions and techniques compound over time, making our codebase cleaner.
Let’s explore some of these topics in this article.
1.) insertIf
In product-grade code, you often define an array with a set of values. However, some values should only appear based on specific conditions.
This is often the case with table columns, where certain columns are displayed based on specific conditions.
For this you can use insertIf
.
Let’s say you have a user overview table, and you want to dynamically control which columns are displayed:
2.) notUndefined
In production-grade code, you should aim for simplicity and type safety.
notUndefined
helps you easily remove undefined values from an array.
Benefits of this are:
Type safety — TypeScript knows the filtered array only contains non-undefined values
Cleaner code — removes the need for type predicates in filter callbacks
Reusability — can be used across the codebase for consistent undefined filtering
3.) sort functions
In production-grade code, you often need to sort various elements.
However, you shouldn’t have to repeatedly look up the definition of functions, such as Day.js’s diff
, to understand how sorting works.
Another useful method is sortAlphabeticalByProperty
, which is widely used throughout our codebase.
4.) Reducing indentation levels as much as possible
In production-grade code, you need to ensure that indentation is at an absolute minimum. This practice makes the code easier to read.
5.) Naming maps
One of the most common mistakes I have observed in our production code is naming maps in this manner: userMap
or callCenterConversationMap
.
Avoid using map
as the name of a variable in production-grade code. This information is not useful, as I typically rely on my IDE to identify the variable’s type.
A better name is userByID
or callCenterConversationByID
. With this name, I instantly understand that the key of a map is the identifier.
When retrieving a value from the map, it also looks much better.
6.) Component Structure
In production-grade code, it is essential to ensure that your React components are well organized.
Components consist of several entities: useMemo
, useCallback
, Event handler, Custom hooks, etc. The order of these entities should be like this:
State declarations (useState)
Refs (useRef)
Memoized values (useMemo)
Memoized callbacks (useCallback)
Custom hooks
Effects (useEffect, useLayoutEffect)
Event handlers and other functions
JSX
You might think that related code should be close together, like memoizedValue
and the useEffect
at the beginning of the component.
Having a clear order in your React component is more effective. As your codebase grows, it becomes challenging to keep related code close together, making it less likely that other engineers will follow this style.
If your custom hooks don’t dependent on any memoized values or callbacks you can also move them to the 3. position after your refs!
7.) flex items do not shrink properly
If you build production-grade front-end code, you need to know flexbox well! While engineers understand the basics of flexbox, they tend not to dig one level deeper and try to compensate for this with a lot of buggy CSS.
One of the most common issues I have seen is that flex items do not shrink properly. Let’s consider an infinite scroll UI:
You need to set the min-height: 0
. Otherwise the flex-item doesn’t shrink properly.
8.) functional vs non-functional
In production-grade code, you should adopt a functional approach.
Non-functional code:
Functional approach:
Keep this in mind for more complex use cases as well, where you might think a functional approach is no longer possible:
Whenever you have a non-functional approach, ask your favorite AI coding assistant if it is possible to transform it into a functional approach.
Here is why:
Final words on cognitive load
When writing code, your primary goal is to minimize cognitive load for others.
While these tips and tricks may seem minor, they compound over time. This is especially true if you work in a globally distributed team where many people contribute to the repository, allowing you to enforce them through pull request reviews.
If your repository is missing any of the items I mentioned earlier, go ahead and add them now! Your teammates will appreciate it.
💡 Want More Tools to Help You Grow?
I love sharing tools and insights that help others grow — both as engineers and as humans.
If you’re enjoying this post, here are two things you shouldn’t miss:
🔧 Medium Insights — A tool I built to explore the analytics behind any Medium author, helping you uncover what works and why.
📅 Substack Note Scheduler — This script allows you to schedule Substack Notes using a Notion database. Basic coding experience is required to set this up. It is free to use :)
📚 Everything I Learned About Life — A Notion doc where I reflect on lessons learned, practical tools I use daily, and content that keeps me growing.
👉 Find all resources(and more) here: Start Here
Great article! I especially appreciate you highlighting these practical tips for cleaner code. From my experience, points 4 and 8, about reducing indentation and embracing functional approaches, are often surprisingly overlooked yet make a huge difference in long-term maintainability. Simple changes in these areas can dramatically improve readability and reduce cognitive load for everyone working on the codebase.